Basics Fundamental of Apex Triggers
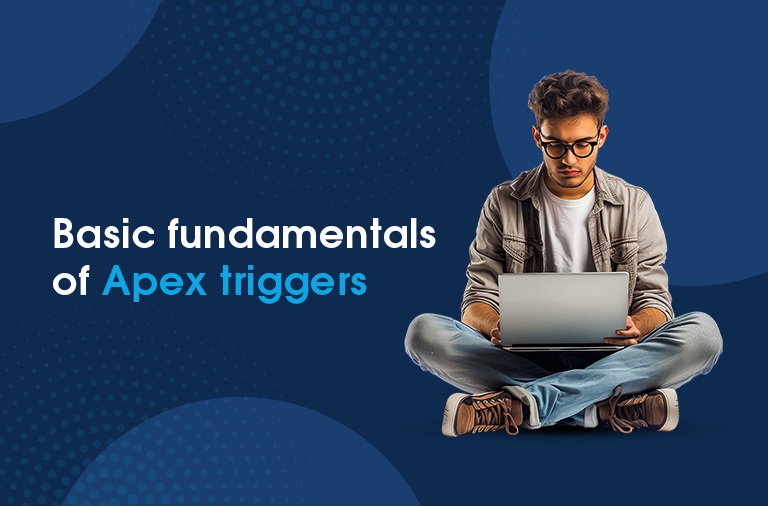
Basic Understanding of Apex Triggers in Salesforce
Apex triggers are powerful tools in Salesforce that automate business processes by allowing you to execute custom actions in response to specific events on records. Whether you’re an experienced developer or new to Salesforce, understanding Apex triggers and how to implement them effectively is crucial for customizing your Salesforce environment. This guide covers what Apex triggers are, when to use them, the differences between Apex triggers and Flows, recursion management, and best practices.
What Are Apex Triggers in Salesforce?
A Salesforce Apex Trigger is a piece of code that automatically executes in response to specific events in a Salesforce database. Triggers allow you to perform custom actions before or after events such as insertions, updates, or deletions of records.
When Should We Use Apex Triggers?
While Salesforce provides various automation tools, here are scenarios where Apex triggers are most suitable:
Complex logic: When logic cannot be handled using declarative tools like workflows or process builders.
DML operations on unrelated objects: When you need to perform actions on objects that don’t have a direct relationship.
Integration with external systems: When you need to interact with external systems in real-time.
Types of Apex Triggers
Apex triggers are classified into two main types based on when they execute relative to the event:
Before triggers: Execute before records are saved to the database. They are used to update or validate records before the changes are committed.
After triggers: Execute after records have been saved to the database. They are used for post-processing tasks such as updating related records, sending emails, or initiating other processes.
Trigger Syntax
Here’s the basic syntax for an Apex trigger:
“`apex
trigger TriggerName on ObjectName (trigger_events) {
// Custom trigger logic
}
“`
ObjectName: The API name of the target object.
trigger_events: The events that trigger the execution (e.g., `before insert, after update`).
Trigger Context Variables
Context variables provide information about the records being processed. These variables are part of the `System.Trigger` class and are essential for building effective triggers:
Trigger.new: A list of new records or new versions of existing records. Used in `insert`, `update`, and `undelete` triggers.
Trigger.old: A list of old versions of records, available in `update` and `delete` triggers.
– `Trigger.newMap`: A map of record IDs to the new versions of records, available in `before update`, `after insert`, `after update`, and `after undelete` triggers.
Trigger.oldMap: A map of record IDs to the old versions of records, available in `update` and `delete` triggers.
Trigger.isExecuting: Returns `true` if the current context is a trigger.
– `Trigger.isInsert`, `Trigger.isUpdate`, `Trigger.isDelete`, `Trigger.isUndelete`: Boolean values indicating the type of operation that triggered the execution.
– `Trigger.isBefore`, `Trigger.isAfter`: Indicate whether the trigger was executed before or after the record was saved.
Trigger.operationType: Returns a `System.TriggerOperation` enum indicating the operation (`BEFORE_INSERT`, `BEFORE_UPDATE`, etc.).
Key Notes:
– In `before` triggers, you can modify `Trigger.new` records, but in `after` triggers, modifying `Trigger.new` results in a runtime exception.
– `Trigger.old` is always read-only.
– You cannot delete records referenced by `Trigger.new`.
Bulk Triggers
Apex triggers are designed to work in bulk, allowing them to handle multiple records simultaneously. This capability ensures that triggers efficiently process large sets of records while adhering to Salesforce’s governor limits, such as the number of DML operations and SOQL queries per transaction.
Bulk Triggers and Governor Limits
DML Limits: Salesforce enforces a limit of 150 DML statements per transaction. Bulk processing allows you to perform operations on multiple records using fewer DML statements.
SOQL Limits: Salesforce allows 100 SOQL queries per transaction. Bulk processing enables you to optimize queries by handling multiple records in a single query.
Best Practices for Apex Triggers
Adhering to best practices ensures that your Apex triggers are efficient, maintainable, and free from common pitfalls:
-
- One Trigger Per Object: Avoid using multiple triggers on the same object to prevent issues with execution order. Instead, consolidate logic into a single trigger and delegate the processing to handler classes.
- Keep Triggers Simple: Delegate complex business logic to helper classes to keep the trigger code clean and focused on invoking the correct logic.
- Always Write Test Classes: Ensure that your triggers have good test coverage to catch edge cases and confirm that your code behaves as expected.
- Avoid Hardcoding: Use custom settings, custom metadata types, or custom labels to avoid hardcoding values in your triggers.
- Bulkify Your Code: Ensure that your trigger can handle bulk operations efficiently to prevent hitting governor limits.
Recursion in Apex Triggers
Recursion is a common issue in Apex triggers where a trigger invokes itself repeatedly, leading to an infinite loop and exceeding governor limits. Understanding and managing recursion is vital to ensure that your triggers perform correctly without unintended side effects.
Common Causes of Recursion
-
- A trigger that updates records, causing the same trigger to fire again.
- Flows or other automation tools that modify records, triggering the same Apex trigger.
How to Avoid Recursion
-
- Static Variables: Use static Boolean flags in an Apex class to control whether a trigger should run.
- Trigger Frameworks: Implement a trigger handler framework that manages recursion and execution flow.
- Check Execution Depth: Use `Limits.getTriggerDepth()` to monitor how deep the recursion goes and stop further execution if necessary.
Difference Between Apex Triggers and Flows
While both Apex triggers and Flows are used to automate processes in Salesforce, they have distinct differences:
Apex Triggers:
-
- Code-Based Automation: Written in Apex, suitable for complex business logic, and offers maximum customization and flexibility.
- Developer-Centric: Requires programming skills and provides full control over logic and execution, including advanced debugging tools.
- Ideal for Complex Logic: Best for scenarios where declarative tools are insufficient.
Flows:
-
- Declarative Automation: Created using a point-and-click interface without the need for code, making it accessible to non-developers.
- Admin-Friendly: Popular among salesforce admins & salesforce consultant in USA for handling standard business processes like creating, updating, or deleting records.
- Visual Design: Designed visually using a flowchart-like interface, making it easier to understand and manage.
- Limited Customization: Suitable for simpler processes where advanced custom logic is not required.
When to Choose Between Apex Triggers and Flows
Choose Apex Triggers: when you need to implement complex business logic, interact with external systems, or require fine-grained control over execution.
Choose Flows: when the process can be handled declaratively without requiring custom code, especially when the business logic is straightforward.
Conclusion
Apex triggers are powerful tools in Salesforce that enable automation of business processes through custom logic triggered by specific events. By understanding when to use them, following best practices, managing recursion effectively, and knowing the differences between Apex triggers and Flows, developers can create scalable, maintainable, and efficient automation solutions within the Salesforce ecosystem. With proper implementation and testing, Apex triggers can significantly enhance the capabilities of your Salesforce environment, driving greater efficiency and business value.
This expanded version covers the additional topics in detail, providing a comprehensive guide to Apex triggers in Salesforce.
related blog
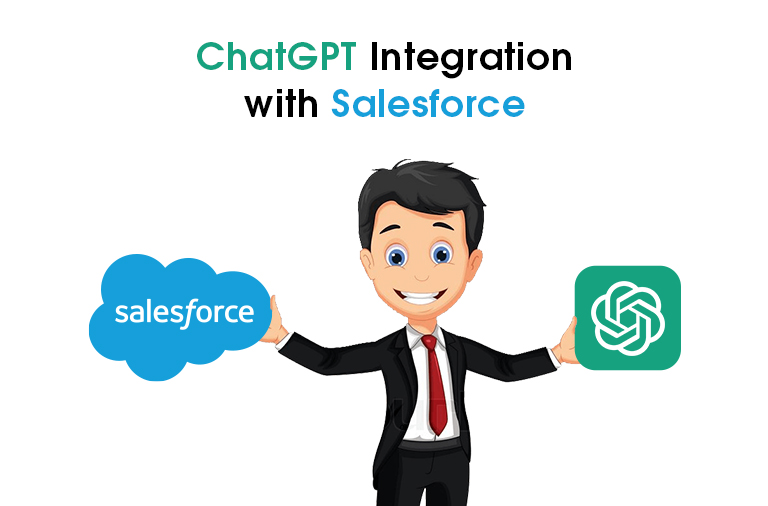
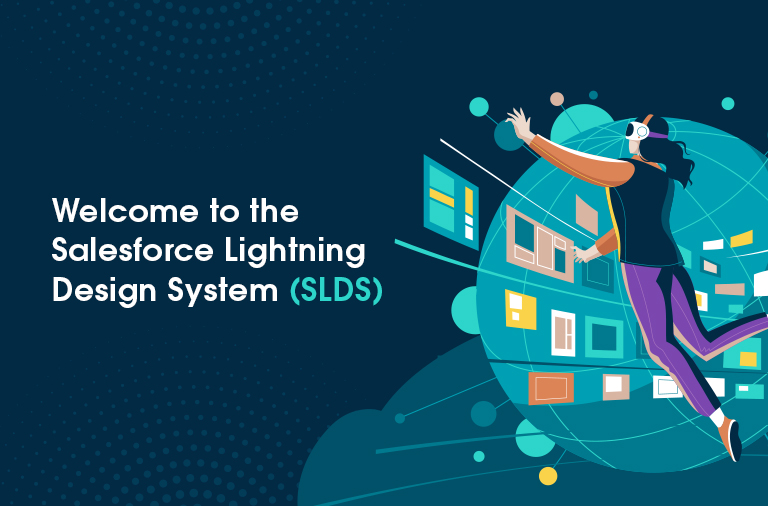
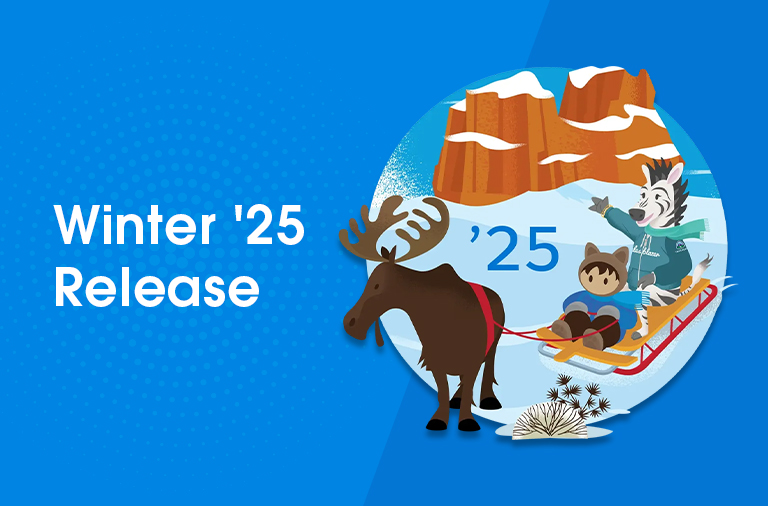